Working with Additional Properties
Adding Additional Properties
Several object types in Cartella implement IExtensibleObject. This interface defines one property.

public interface IExtensibleObject : ICartellaObject { IAdditionalProperties AdditionalProperties { get; } }
IAdditionalProperties defines several properties

public interface IAdditionalProperties { int Count { get; } bool ContainsKey(string key); bool TryGetValue(string key, out ICustomFieldInfo value); ICollection<string> Keys { get; } ICollection<ICustomFieldInfo> Values { get; } IEnumerator<KeyValuePair<string, ICustomFieldInfo="">> GetEnumerator(); ICustomFieldInfo this[string key] { get; } }
Additional Properties can be added to objects by carefully adding records to the Database, but it is recommended to use the Standard Cartella UI as shown below. Navigate to an Edit Form for the type of object that you want to add fields to. Click the "Manage Fields" button.
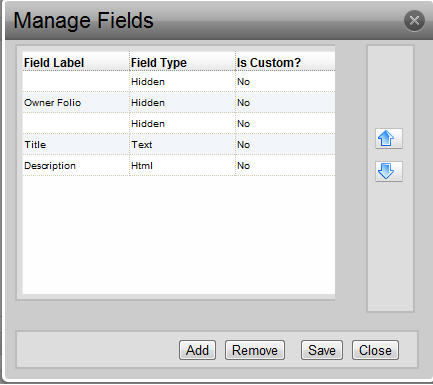
![]() |
---|
Only site Admins can get to this UI |
Field Name will be the Name displayed on the Edit form. Field Unique ID is the key that will be used to retrieve the value of the property. Field type can be one of several choices.
Document - This is an asset with no preview.
Checkbox - A boolean property
Date - A nullable DateTime Field. The edit form will render a date picker. This field could be used to also store a time, however you would need to implement this.
HTML - This is stored as a string. For a field of this type the Edit Form will render a TinyMCE Editor
Image - Simillar to Document except that the Edit form will render an image maniuplator.
Text - A simple string field
Hidden - This can be used to store information that you do not want to appear on the edit form.
Caution
If you will be modifying a hidden field's value in the custom hooks file, it is advisable to manually remove the record in Table_EditFormDefinition_Fields for this field. This will remove the field from the edit form entirely, but leave it editable on the object via custom code.
Retrieving Additional Property Values
Because you need to know the string key to retrieve the property value and you may want to do additional checks for null values, it is recommended that you wrap retrieving the values in extension functions.

public static string GetAdditionalInformationString(this HtmlHelper helper, IEntity ent, String key) { string propVal = string.empty; if (ent != null) { IAdditionalProperties props = ent.AdditionalProperties; if (props[key] != null && !string.IsNullOrEmpty(props[key].ValueStr)) propVal = props[key].ValueStr; } return propVal; } public static string GetAdditionalInformationString(this HtmlHelper helper, IUser user, String key) { string propVal = string.empty; if (user != null && !user.IsAnonymous) //anon users don't have additional properties { IAdditionalProperties props = user.AdditionalProperties; if (props[key] != null && !string.IsNullOrEmpty(props[key].ValueStr)) propVal = props[key].ValueStr; } return propVal; }
The above methods should only be used with Text or HTML fields. Here is an example of how to retrieve a Document Property

string docUrl = string.Empty; if (item.AdditionalProperties.ContainsKey("doc")) { DocumentAsset doc = (DocumentAsset)item.AdditionalProperties["doc"].Value; docUrl = doc.Url; }
A similar method could be used for an image, except that you would cast the value into a Cartella.Classes.ImageAsset.