By default, Cartella uses its internal system to authenticating users. This doesn't have to be the only way. Cartella allows custom authentication plug-ins to be created. For example, we can have Cartella authenticated against Active Directory.
We will create an Authenticator Plug-in called "LDAP Authenticator".
Create a new Class Library project with Visual Studio 2008. Make sure it is using .NET framework 3.5. Add CartellaBase.dll and Common.dll from Cartella as references.
Create a new class called "LDAPAuthenticator", having it inherit the interface IAuthenticator. Implement the interface. Here we have the raw version:

using System; using System.Collections.Generic; using System.Linq; using System.Text; using Cartella.Interfaces; namespace Cartella.Extension { public class LDAPAuthenticator : IAuthenticator { #region IAuthenticator Members public bool Authenticate(IUser user, string password) { ///////////////////////////////////////////////// /// Authentication details goes here ///////////////////////////////////////////////// return authenticated; } public string Description { get { return "Mock up LDAP Authenticator"; } } public string FriendlyName { get { return "LDAP Authenticator"; } } #endregion } }
Fill in the details of the class. Build it, and deploy it in the "bin" folder under the Cartella web application location.
Now when you create and new user, in the user creation form, you will see the authenticator listed:
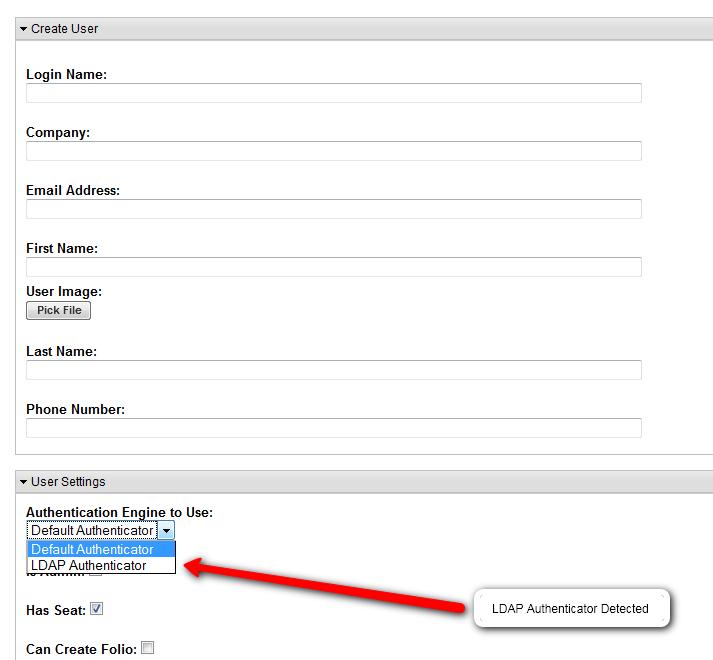
This way you can select LDAP authenticator for this user. In the future, when authenticating this user, LDAP authenticator will be used.
![]() |
---|
This way you can select LDAP authenticator for this user. In the future, when authenticating this user, LDAP authenticator will be used.
|
![]() |
---|
If the Cartella version is changed, make sure to re-import the references for the authenticator plug-in project, recompile and deploy the dll. Otherwise, the authenticator plug-in will not be detected. |