Cartella provides a full-scale Asset Management system, with a plug-in based storage provider, a version control system, and multiple-member support. The Asset system is fully exposed through the Cartella API.
Structure of an Asset
What sets the Cartella asset system apart from other systems is the following feature set:
- Each Asset can contain multiple members.
- Each Asset Member can contain multiple versions, each version with its own binary file.
- Asset itself is also versioned, which consists of a combination of binary versions from members.
- A plug-in based automatic conversion system can be put in place to automatically convert one member to another member.
- Interface to the Asset system is simple and easy to use.
Graphical View of Asset Management
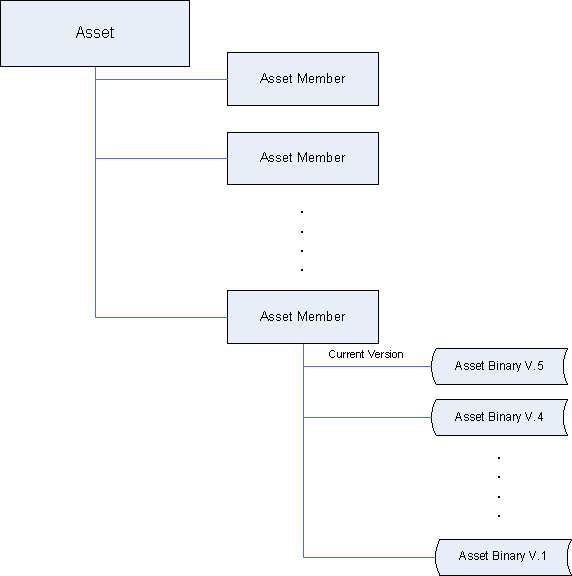
Assets that are properties of objects
Many Cartella objects have an Asset property:
CopyC#
IAsset userImage = user.Image;
Asset objects are retrieved from the CartellaObject instance that owns it.
The most common use of an Asset object is to retrieve its URL:
CopyC#
string userImageUrl = userImage.Url;
![]() |
---|
The URL of an Asset is a combination of an Asset Binary unique ID and an Asset Member filename and extension. It is in the structure of Asset/[binaryID]/[member file name].[member file extension]. The critical part of the URL is the Asset Binary unique ID. It will be used by the AssetManager to retrieve the Asset Binary, thus providing the content of the Asset Binary. The example below demonstrates how to use the URL to stream Asset Binary content as an HTTP Response, in a web application: ![]() string assetBinaryId = processAssetUrl(assetUrl); IAssetSystemManager assetManager = siteManager.AssetManager; IAssetBinary binary = assetManager.SelectSingleAssetBinary(assetBinaryId); if (binary != null) { Stream binaryStream = binary.File; string contentType = (binary.DefaultAdditionalProperties != null && binary.DefaultAdditionalProperties.ContainsKey("MimeType")) ? assetBinary.DefaultAdditionalProperties["MimeType"] : "application/octet-stream"; using (binaryStream) { byte[] buffer = new byte[4096]; while (true) { int read = binaryStream.Read(buffer, 0, buffer.Length); if (read == 0) break; response.OutputStream.Write(buffer, 0, read); } } response.Flush(); response.End(); } |
Managing Assets, Asset Members, and Asset Binaries
The Cartella Asset Management system allow Assets, Asset Members, and Asset Binaries to be created, updated, and deleted via the Cartella API.
Please refer to IAssetSystemManager section for more details

//retrieve the AssetSystemManager instance from the SiteManager IAssetSystemManager assetManager = siteManager.AssetManager; //retrieve asset from user IAsset asset = user.Image; //cast the asset to ImageAsset type ImageAsset image = asset as ImageAsset; //Linq statement to retrieve all member urls with jpg as extension var members = from assetMember in image.Members let extension = assetMember.FileExtension.ToLower() where extension == "jpg" select assetMember; IAssetMember member1 = members.FirstOrDefault(); if (member1 != null) { //get all previous version before year 2009 var versions = from bin in member1.PreviousVersions where bin.CreationDate.Year < 2009 select bin; List<IAssetBinary> binariesBefore2009 = versions.ToList(); //add a new version to this member member1.AddVersion(FileStream, "png", new Dictionary<string, string>() { {"alt", "Cartella Log"}, {"target", "_blank"} }); }