Prerequisites: Administrators must navigate to the ICE list element's schema in
and select the list element's
Grouped in expanded
XML checkbox.
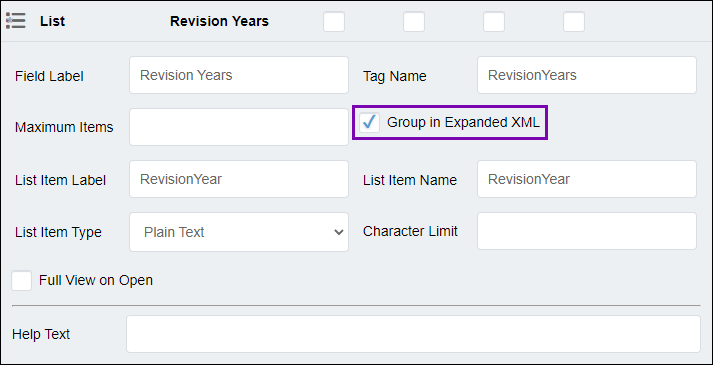
For content contributors to edit list field elements via In-Context Editing (ICE) mode, the
system requires developers to first create a custom ASP.Net view to associate with
the list element.
To create a custom view template to work with the list element in
ICE:
- Open your project in Visual Studio.
- Use the following code samples to help you model your own custom ICE
view.
- Home.cshtml: Calls partial view that contains ICE
helper.
@model Ingeniux.Runtime.CMSPageRequest
@using Ingeniux.Runtime
@using System.Data.SqlClient;
<!DOCTYPE html>
<html lang="en">
<head>
...
</head>
<body>
<!-- Main -->
...
<section id="main" class="wrapper">
<div class="container">
@Html.Partial("editable/RevisionYears", Model.Element("RevisionYears"))
</div>
</section>
...
</body>
</html>
- RevisionYears.cshtml: Partial view includes ICE helper
(i.e.,
@_Helpers.RenderICEAtrribute()
).
@model Ingeniux.Runtime.ICMSElement
@using Ingeniux.Runtime
@if (Model != null || Model.EditMode)
{
IEnumerable<ICMSElement> revisionYears = Model.Elements("RevisionYear");
<div @_Helpers.RenderICEAttribute(Model)>
@if (revisionYears != null && revisionYears.Count() > 0)
{
<p>
@foreach (ICMSElement year in revisionYears)
{
@Html.Partial("editable/_DefaultText", year)
<br />
}
</p>
}
</div>
}
- _DefaultText.cshtml: Partial view renders ICE list
Note
The @placeholderText
value displays in ICE
mode if items in the list do not contain values. The
@placeholderText
ensures that at least one list item
is always viewable and clickable in ICE
mode.
@model Ingeniux.Runtime.ICMSElement
@using Ingeniux.Runtime
@if (Model != null && (!String.IsNullOrWhiteSpace(Model.Value) || Model.EditMode))
{
var placeholderText = ViewData["Placeholder"] != null ? ViewData["Placeholder"].ToString() : String.Empty;
var value = Model.Value;
<span @_Helpers.RenderICEAttribute(Model)>
@if (!String.IsNullOrWhiteSpace(value))
{
@Html.Raw(value)
}
else if (Mode.EditMode && !String.IsNullOrWhiteSpace(placeholderText))
{
@placeholderText <text>Placeholder</text>
}
</span>
}